The HelpDesk RESTful API is a transformation project designed to consolidate the knowledge acquired in the Spring Framework. The API serves as the backend for a ticket management application. The project utilizes Spring Boot, Spring Web, Spring Data, Spring Validation, Spring Security, Spring Test, JWT, and OAuth 2 Resource Server. The application follows best practices for RESTful API development, consistently returning appropriate HTTP status codes for each operation.
The application features authentication and authorization controls, with areas accessible only to authenticated users with specific roles. Each user is assigned a role upon registration, which can be a client, technician, or administrator:
- Users with the client role can register in the system and view ticket information.
- Users with the technician role can create new tickets.
- Users with the administrator role can register new technicians.
In addition to user roles, the application has two startup profiles. In the test profile, the application uses an H2 database; in the dev profile, it uses a MySQL database. The startup profile can be easily changed by modifying the spring.profiles.active property in the application.properties configuration file.
The HelpDesk RESTful API excels in exception handling, providing the return code, error type, error description, and invalid attributes when an error occurs.
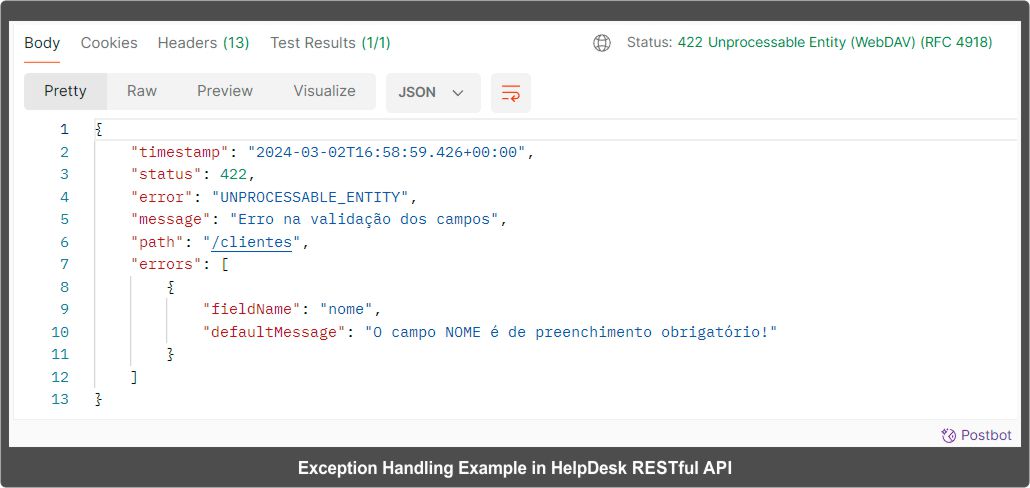
The project features detailed unit tests, along with an initial load of test data that allows easy testing in Postman as soon as the application is initialized.
Built between December 7th, 2022, and February 12th, 2024, one year and three months, it ultimately consumed 185 hours of work divided into four stages:
- Based on the course Angular + Spring Boot Training (Udemy - Valdir Cezar)
Stage 1 defined the project concept and created the initial part of the application code:
- Model layer
- Entity mapping with JPA
- DTO layer
- Controller layer
- Service layer
- Repository layer
- Enumerations
- Application startup profiles (dev and test)
- Transformation
Stage 2 marked the beginning of the project's transformation:
-
In the original project, the application uses only one DTO object for each model. Consequently, the controller layer does not return the DTO of the registered or updated Client or Technician in the system — it only returns the HTTP status codes of the operation. This is because returning the DTO also means returning its password — even if encrypted, returning the user's password is a serious security breach.
The transformed project uses one DTO object for the request and another for the response. Thus, ClientRequestDTO and TechnicianRequestDTO contain the password attribute, while ClientResponseDTO and TechnicianResponseDTO do not. This allows the return of the registered or updated Client or Technician DTO in the system without exposing their password.
-
In the original project, when creating or updating a Client, it is possible to send an array of roles in the request. This allows to create or modify a Client so that they accumulate the roles of client, technician, and administrator. This constitutes a serious security breach as, according to business rules, the client can perform or modify their registration in the system — thus, the client can grant themselves super privileges.
In the transformed project, the service handling the request does not allow the registered or modified Client to accumulate roles. Thus, even if an array of roles is sent in the request, at the end of the operation, the Client will only have the client role.
-
In the original project, every registered Technician automatically receives the client and technician roles — however, this is done in the DTO layer.
In the transformed project, this is handled by the service — thus separating the data transport layer from the business rules.
- In the transformed project, the enumerations layer were moved out of the domain layer and placed at the root of the project — making them easier to find.
- In the transformed project, the domain layer was renamed to model — making it easier to find.
- In the transformed project, the UserSS class (which implements UserDetails) was moved from the security folder (deleted from the application) to the model folder — along with the other system models.
-
In the original project, the application uses only one DTO object for each model. Consequently, the controller layer does not return the DTO of the registered or updated Client or Technician in the system — it only returns the HTTP status codes of the operation. This is because returning the DTO also means returning its password — even if encrypted, returning the user's password is a serious security breach.
- Based on the video trilogy Spring Security & JSON Web Tokens (YouTube - Dan Vega)
Stage 3 implemented authentication with Spring Security + JWT and authorization with OAuth 2 Resource Server.
- Based on the course Practical Automated Testing with Spring Boot (Udemy - Giuliana Silva Bezerra)
Stage 4 developed:
- Test scenario diagrams — elegantly crafted in Corel Draw and displayed in the project's image gallery.
- Unit tests with Spring Boot.
This stage also defined the appropriate HTTP status codes for each type of operation — including exception handling.
To Learn More
- Angular + Spring Boot Training Course - Udemy/Valdir Cezar
- How to secure your Spring Boot REST APIs with JSON Web Tokens - YouTube/Dan Vega
- Spring Security JWT: How to authenticate with a username and password - YouTube/Dan Vega
- Secure your REST APIs with Spring Security & Symmetric Key Encryption - YouTube/Dan Vega
- Practical Automated Testing with Spring Boot Course - Udemy/Giuliana Silva Bezerra